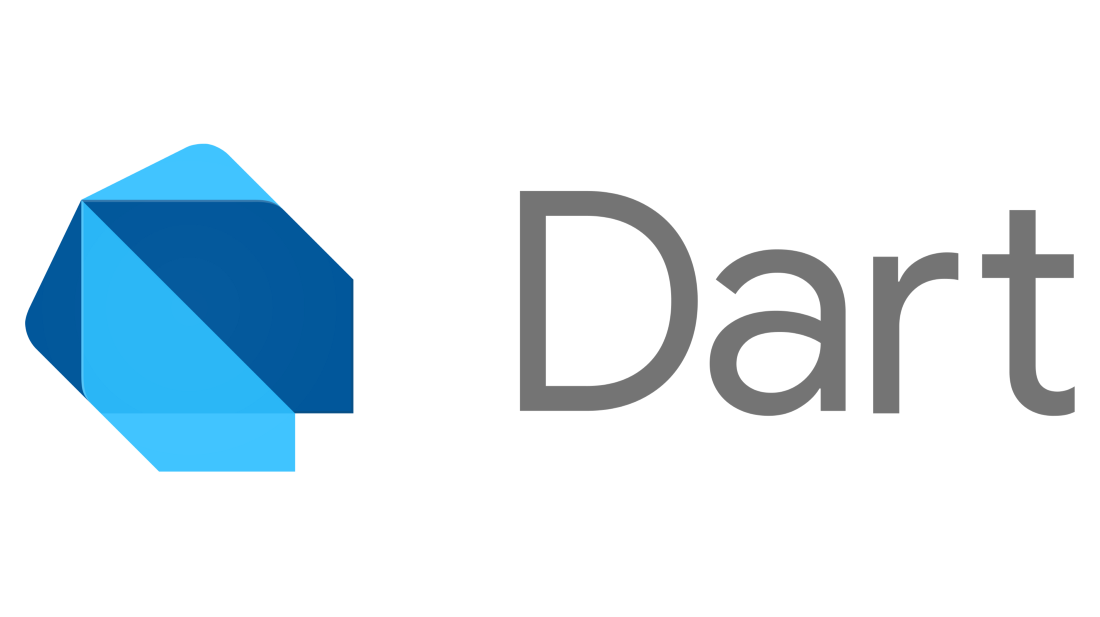
Week 9: More on Dart!
Learn to develop with Dart!
Dart Challenge
We have a challenge on Dart programming language this week. Dart, developed by Google, it can be used to make web applications and mobile applications. It supports both the client and server side. It can also be used with Flutter.
Introduction
Dart is a versatile and powerful programming language developed by Google. It is used for building web, mobile, and server applications. Dart is particularly known for its use with the Flutter framework, making it a popular choice for cross-platform development.
Dart Programming Language - Key Features
Dart is a versatile and powerful programming language developed by Google. It is used for building web, mobile, and server applications. Dart is particularly known for its integration with the Flutter framework, making it a popular choice for cross-platform development.
Key Features of Dart
1. Ahead-of-Time (AOT) Compilation
Dart supports AOT (Ahead-of-Time) compilation, which converts Dart code into highly optimized native machine code before execution. This significantly improves performance, making Dart a great choice for mobile and web applications.
2. Just-in-Time (JIT) Compilation
For fast development cycles, Dart provides JIT (Just-in-Time) compilation. This allows for features like hot reload in Flutter, enabling developers to see changes instantly without restarting the app.
3. Strongly Typed with Type Inference
Dart has a sound type system that ensures type safety while allowing for flexibility through type inference. This means that developers can omit explicit type declarations in many cases, making code cleaner while maintaining safety.
4. Asynchronous Programming
Dart excels in handling asynchronous operations using:
Future
andasync/await
, which make working with asynchronous code more readable.Stream
for handling multiple asynchronous events over time, such as real-time updates.
5. Garbage Collection and Memory Management
Dart includes automatic memory management with an efficient garbage collector. This ensures that unused memory is freed up, preventing memory leaks and improving application performance.
6. Platform Independence
Dart runs on multiple platforms, including:
- Client-side: Web and mobile applications using Flutter.
- Server-side: Backend services using frameworks like Dart Frog or Aqueduct.
- Embedded Systems: With Dart Native, it can be used for embedded development.
7. Object-Oriented Programming (OOP)
Dart is an object-oriented language with support for:
- Classes and Objects for structuring code.
- Interfaces and Abstract Classes to enforce structured development.
- Mixins and Extensions to allow for code reuse without traditional inheritance.
8. Rich Standard Library
Dart comes with a built-in standard library that provides:
- Core functionalities like data structures (Lists, Maps, Sets).
- File and network handling (HTTP, WebSockets).
- Utilities for math, dates, and string manipulation.
9. Null Safety
Dart enforces null safety, reducing the risk of null reference errors. This feature ensures that variables cannot hold null unless explicitly allowed, making code more robust and preventing unexpected crashes.
10. High Performance
Dart applications run efficiently due to:
- Native compilation for optimized execution.
- Low-latency garbage collection for smooth performance.
- Optimized JIT and AOT compilation techniques.
11. Easy Interoperability
Dart can easily interoperate with:
- JavaScript for web applications using
dart2js
compiler. - C/C++ via FFI (Foreign Function Interface) for high-performance applications.
Challenges
1. Basic Calculator (100 points)
Implement a basic calculator that performs addition, subtraction, multiplication, and division.
Fill in the Blanks:
import 'dart:io';
void main() {
print("Enter first number:");
double num1 = ???
print("Enter operator (+, -, *, /):");
String op = ???
print("Enter second number:");
double num2 = ???
double result;
switch (op) {
???
}
print("Result: \$result");
}
2. Unit Converter (100 points)
Create a terminal-based unit converter that converts miles to meters.
Fill in the Blanks:
import 'dart:io';
void main() {
`//Parse input
//Some unit conversion
//print output
}
3. Chat Application (200 points)
Create a real-time chat application where users can send messages to each other.
Cool Dart Features
1. Asynchronous Programming
Future<String> fetchData() async {
await Future.delayed(Duration(seconds: 2));
return "Data loaded";
}
void main() async {
print("Fetching data...");
String data = await fetchData();
print(data);
}
2. Extension Methods
extension StringExtensions on String {
String get reversed => split('').reversed.join();
}
void main() {
print("Dart".reversed); // Output: traD
}
3. Null Safety
void greet(String? name) {
print("Hello, \${name ?? 'Guest'}");
}
void main() {
greet(null); // Output: Hello, Guest
greet("Alice"); // Output: Hello, Alice
}
Dart is a powerful and efficient language for various applications, from simple terminal programs to full-fledged cross-platform apps. These challenges are a great way to sharpen your skills and explore the capabilities of Dart!